So now in our third act, we’re ready to write out a script for our game. Our Node and Stack classes are written, and ready to be imported. Let’s initiate our game by welcoming the player with a print statement — Let’s play Towers of Hanoi!
The first thing we should do is build the infrastructure of Hanoi Towers. There are three stacks, and some disks to move around. We’ll let the player decide the number of disks, so let’s just set up the three stacks first. Thankfully I already have a Stack class for that. I’ll make three of them: left, middle, and right respectfully, and put them into a list variable so they’re easier to keep track of. I’ll then create a disk variable for the number of disks set by user input.
However, the game should have at least 3 disks to make it interesting, so I’ll set a while loop to keep asking the user for an input till it’s at least 3 disks.

So far so good. All the disks begin on the left stack, so let’s create a for loop to push() the disks onto it. The bigger disks should go on the bottom so I’ll run the for loop with a reverse range.
Also, just for kicks, I’ll calculate the minimal numbers of moves that the player could finish the game in using the formula: (2 ** num_disks) — 1.

Okay, so to get this game going, I’ll need a helper function that’ll loop through the options (left stack, middle stack, right stack), and ask for an input from the player. If the input matches one of the choices I’ll loop through the stack choices, and return that stack.

Alright, so let’s get to the meaty part of the game. I’ll run a while loop until all the disks are on the right stack. So while the game is going (the right_stack doesn’t equal the number of disks) I’ll print the status of the game looping through the stacks using the print_items() function.
And while that is true I’ll first ask “Which stack do you want to move from?”. I’ll then store the answer in a from_stack variable, and use my helper function to make sure the logic for the answer is possible. I’ll also do the same for the to_stack input.
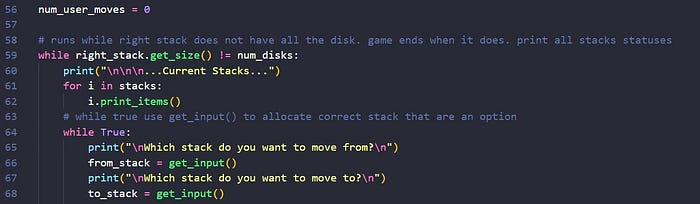
If the from_stack variable has nothing in it I’ll ask for re-entry of input as it’s an invalid move.
I also want to verify that the input for the to_stack either has nothing in it or that the from_stack disk is smaller than the to_stack disk. If it meets those requirements I’ll pop() the disk from from_stack, and push() it to the to_stack, and increase the num_user_moves variable I set up to keep track of the number of moves. I’ll then break out of the cycle and run the while True loop again if the first while condition hasn’t been met (all disk to the right_stack).
Lastly, I’ll make sure that there is an else statement if the first two if-conditions aren’t met, because the move was invalid.

That’s it. It’s pretty simple. I always find the trickiest parts being the embedded while loops, and making sure you break out of it properly. But it’s all there. Definitely take the time to make sure you understand it as it really helps your fundamentals.
Thanks for following along on this little game of a journey, and I hope it helped break down how easy programming in Python can be!