If you’re interested in writing Python, then at some point you’ve probably wondered how to automate parts of your life, and have your script run automatically on its own.
In this article, I’m going to explain a simple python script to send emails, and how you can have that email sent automatically without ever having to touch the code again.
First, let’s write some code. In Python we’ll need to import a couple of libraries to make the magic work: smtplib for our email functionality. This will connect to our email server (in this case a Gmail account).
To easily organize our email structure I’ll use the EmailMessage function from the email.message library, and the datetime library to easily access the current date so I know I’m sending my emails out on the correct day. I’m also going to populate a simple array of contacts in a new file contactList and import that in. These will be the folks I want to email, and as the list grows it’ll be easier to manage in a different file. Whenever possible always break your code into modules. It’s a good habit to establish.
import contactList
import smtplib
from email.message import EmailMessage
from datetime import date
For this to work, I’ll be sending the emails from my personal account. I’ll import my username and password from a separate text file for safekeeping. You can just put that information directly into your script if you don’t ever plan to make it public.
Our Python class is going to be a happy birthday message we’ll send to friends. I’ll initialize it with some basic parameters like name, email, date, sex, and subject. I’ll send two different emails depending if the recipient is male or female.
class birthdayEmail: def __init__(self, name, email, date, sex, subject="Happy Birthday!"): self.name = name self.email = email self.subject = subject self.date = date self.sex = sex.lower()
Next, I’ll want a function to tell me the date. I don’t care for the year so I can leave that out.
def monthDay(self):
todayDate = date.today().strftime(’%m/%d’)
return todayDate
Okay, so for the email function I’ll initialize the EmailMessage into a msg variable, and from there load the email’s subject, from, to, and BCC fields.
def sendEmail(self):
msg = EmailMessage()sg['Subject'] = self.subject
msg['From'] = EMAIL_ADDRESS
msg['To'] = self.email
msg['BCC'] = "email@gmail.com"
if(self.sex == 'm') and (self.date == self.monthDay()):
msg.set_content("Happy birthday man {0}".format(self.name))
elif(self.sex == 'f') and (self.date == self.monthDay()):
msg.set_content("Happy birthday lady {0}."format(self.name))
You can see I used the set_content() function to add the message of my email. Now that I have my email message ready I’ll use the smtplib library to do the heavy lifting of calling my Gmail account and sending the email. Do note you will have to change your Gmail account settings to something less secure so it’s a good idea to use a secondary account if you have one.
with smtplib.SMTP_SSL('smtp.gmail.com', 465) as smtp:
smtp.login(EMAIL_ADDRESS, EMAIL_PWD)
smtp.send_message(msg)
Great your class is all set up, now just loop over your contactList array and run your script. But how do we set this up on your PC to run it every day?
Your windows PC already has an app for that known as Task Scheduler. Search for it and you should see:

Here we’ll be making all the changes. Under the Actions sidebar select ‘Create Task’.

Fill in the name, and description as you like. In the Security Options, select ‘Run whether user is logged on or not’, ‘Run with highest privileges’ and ‘Hidden’.

Go ahead and enter the Triggers tab above, and here we can set the schedule. I’d select Daily under settings, and make sure ‘Enabled’ is checked at the bottom.
Next, under the Actions tab find the script with the browse… button, and in the Add arguments box, enter the name of the file, and the path of the file in the start box.
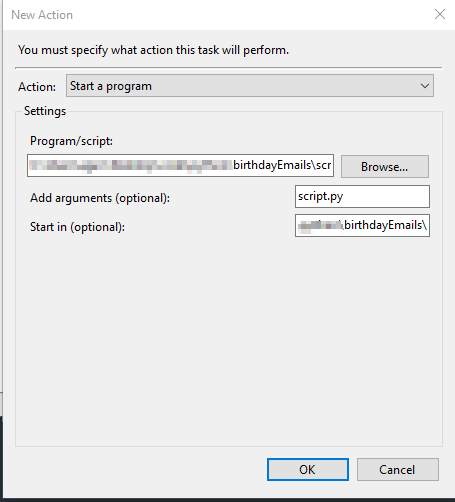
Under the Conditions tab, I’d deselect the ‘Start the task only if the computer is on AC power’, select ‘Wake the computer to run this task’, and the Network box because I will need an internet connection to send out an email. Then we’re done, but feel free to check out the settings tab.
Great. Now, from there, your script will run, and if you have a friend’s birthday on that date, it will be automatically sent out! Way to be a good friend ^*^.